Common Data Processing and Augmentation
When processing data of different categories like image, text and audio, some common data processing and augmentation operations are required.
MindSpore provides the transforms
module for common data augmentation.
The following sections will take the CIFAR-10 dataset and the MNIST dataset as examples to briefly introduce some examples for the common data augmentation operations. For more common data augmentation operations, see API documentation of mindspore.dataset.transforms.
Load Image Data
The following sample code downloads and decompresses the CIFAR-10 dataset and the MNIST dataset to the specified locations, respectively. This code is expected to take three to five minutes to execute when the network is in good condition.
[1]:
import os
from mindvision.dataset import DownLoad
dl_path_cifar10 = "./datasets"
dl_url_cifar10 = "https://mindspore-website.obs.cn-north-4.myhuaweicloud.com/notebook/datasets/cifar-10-binary.tar.gz"
dl = DownLoad()
# Download the CIFAR-10 dataset and unzip it
dl.download_and_extract_archive(url=dl_url_cifar10, download_path=dl_path_cifar10)
# MNIST dataset save path
dl_path_mnist = "./mnist"
dl_url_mnist_labels = "http://yann.lecun.com/exdb/mnist/train-labels-idx1-ubyte.gz"
dl_url_mnist_images = "http://yann.lecun.com/exdb/mnist/train-images-idx3-ubyte.gz"
# Download the MNIST dataset and unzip it
dl.download_and_extract_archive(url=dl_url_mnist_labels, download_path=dl_path_mnist)
dl.download_and_extract_archive(url=dl_url_mnist_images, download_path=dl_path_mnist)
image_gz = "./mnist/train-images-idx3-ubyte.gz"
label_gz = "./mnist/train-labels-idx1-ubyte.gz"
# Delete compressed files
if os.path.exists(image_gz):
os.remove(image_gz)
if os.path.exists(label_gz):
os.remove(label_gz)
Use the mindspore.dataset.Cifar10Dataset interface to load the CIFAR-10 data, and use the mindspore.dataset.MnistDataset interface to load the MNIST data. The example code is as follows:
[2]:
import matplotlib.pyplot as plt
import mindspore.dataset as ds
%matplotlib inline
DATA_DIR_MNIST = "./mnist/"
DATA_DIR_CIFAR10 = "./datasets/cifar-10-batches-bin/"
ds.config.set_seed(1)
# Load the dataset and select 4 images
dataset_cifar10 = ds.Cifar10Dataset(DATA_DIR_CIFAR10, num_samples=4)
dataset_mnist = ds.MnistDataset(DATA_DIR_MNIST, num_samples=4)
def printDataset(dataset_list, name_list):
"""Show dataset"""
dataset_sizes = []
for dataset in dataset_list:
dataset_sizes.append(dataset.get_dataset_size())
row = len(dataset_list) # Displayed number of rows
column = max(dataset_sizes) # Displayed number of columns
pos = 1
for i in range(row):
for data in dataset_list[i].create_dict_iterator(output_numpy=True):
plt.subplot(row, column, pos) # Display location
plt.imshow(data['image'].squeeze(), cmap=plt.cm.gray) # Display content
plt.title(data['label']) # Show title
print(name_list[i], " shape:", data['image'].shape, "label:", data['label'])
pos = pos + 1
pos = column * (i + 1) + 1
printDataset([dataset_cifar10, dataset_mnist], ["CIFAR-10", "MNIST"])
CIFAR-10 shape: (32, 32, 3) label: 9
CIFAR-10 shape: (32, 32, 3) label: 2
CIFAR-10 shape: (32, 32, 3) label: 0
CIFAR-10 shape: (32, 32, 3) label: 8
MNIST shape: (28, 28, 1) label: 7
MNIST shape: (28, 28, 1) label: 2
MNIST shape: (28, 28, 1) label: 4
MNIST shape: (28, 28, 1) label: 4
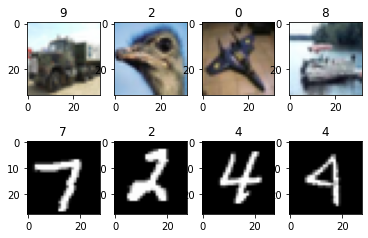
mindspore.dataset.transforms module
The transforms
module supports common data augmentations. Some operations are implemented in C++ to provide high performance. Other operations are implemented in Python including using NumPy.
Compose
The Compose
operation receives a list of transforms operations and composes it into a single transform.
The following example first loads the CIFAR-10 dataset [1], then defines decoding, scaling and data type conversion operations at the same time, and acts on the loaded image. It finally outputs the processed image shape and corresponding label, and performs the image processing on the images.
[7]:
import matplotlib.pyplot as plt
import mindspore.dataset as ds
import mindspore.dataset.vision as vision
from mindspore.dataset.transforms import Compose
from PIL import Image
%matplotlib inline
ds.config.set_seed(8)
# CIFAR-10 dataset loading path
DATA_DIR = "./datasets/cifar-10-batches-bin/"
# Load the CIFAR-10 dataset and select 5 images
dataset1 = ds.Cifar10Dataset(DATA_DIR, num_samples=5, shuffle=True)
def decode(image):
"""Define the decoding function"""
return Image.fromarray(image)
# Define the list of transforms
transforms_list = [
decode,
vision.Resize(size=(200, 200)),
vision.ToTensor()
]
# Apply the operations in the transforms list to the dataset images through the Compose operation
compose_trans = Compose(transforms_list)
dataset2 = dataset1.map(operations=compose_trans, input_columns=["image"])
# The shape and label of the image after printing the data augmentation operations
image_list, label_list = [], []
for data in dataset2.create_dict_iterator():
image_list.append(data['image'])
label_list.append(data['label'])
print("Transformed image Shape:", data['image'].shape, ", Transformed label:", data['label'])
num_samples = len(image_list)
for i in range(num_samples):
plt.subplot(1, len(image_list), i + 1)
plt.imshow(image_list[i].asnumpy().transpose(1, 2, 0))
plt.title(label_list[i].asnumpy())
Transformed image Shape: (3, 200, 200) , Transformed label: 4
Transformed image Shape: (3, 200, 200) , Transformed label: 9
Transformed image Shape: (3, 200, 200) , Transformed label: 6
Transformed image Shape: (3, 200, 200) , Transformed label: 5
Transformed image Shape: (3, 200, 200) , Transformed label: 7
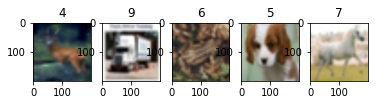
As can be seen from the above printing and displayed picture results, after the data augmentation operations in the transforms list, the picture label has not changed, the shape has changed, and the resolution has been scaled to 200×200.
Deprecated c_transforms
and py_transforms
Beginning in version 1.8 of MindSpore, the following common data transforms modules are deprecated:
mindspore.dataset.transforms.c_transforms
mindspore.dataset.transforms.py_transforms
One should use the following unified module for which the underlying implementation may be C++ code and/or Python code:
Special Attention
When upgrading from the deprecated c_transforms
or py_transforms
modules to the unified modules, the same operation that was used in c_transforms
or py_transforms
can be used with no changes in operation name or input arguments.
Except for the following cases:
From deprecated mindspore.dataset.transforms.py_transforms
, when using unified mindspore.dataset.transforms
:
Replace
OneHotOp
from with unified operation nameOneHot
Please notice that when the operation throws an error, the error message provided from the deprecated operation may be different from the error message provided from the unified operation.
References
[1] Alex Krizhevsky. Learning_Multiple Layers of Features from Tiny Images.
[2] Y. LeCun, L. Bottou, Y. Bengio, and P. Haffner. Gradient-based learning applied to document recognition.
The sample code in this chapter relies on third-party support package
matplotlib
, which can be installed using the commandpip install matplotlib
. If this document is run underNotebook
, you need to restart the kernel after installation to execute subsequent code.